Tracecut Functions
Functions | |
enum tc_error | tc_error_code (struct tc_tracecut *tc) |
void | tc_reset_error (struct tc_tracecut *tc) |
enum tc_error | tc_add_param (struct tc_tracecut *tc, const char *name, const struct aop_type *type) |
enum tc_error | tc_add_call_symbol (struct tc_tracecut *tc, const char *name, const char *func_name, enum aop_insert_location location) |
enum tc_error | tc_bind_to_call_param (struct tc_tracecut *tc, const char *param_name, const char *symbol_name, int call_param_index) |
enum tc_error | tc_bind_to_return_value (struct tc_tracecut *tc, const char *param_name, const char *symbol_name) |
enum tc_error | tc_declare_call_symbol (struct tc_tracecut *tc, const char *name, const char *declaration, enum aop_insert_location location) |
enum tc_error | tc_add_rule (struct tc_tracecut *tc, const char *specification) |
struct tc_tracecut * | tc_create_tracecut (void) |
void | tc_free_tracecut (struct tc_tracecut *tc) |
void | tc_insert_tracecut_init_advice (struct aop_joinpoint *jp) |
void | tc_set_main_function (const char *func_name) |
void | tc_register_tracecut_pass (void) |
Detailed Description
In InterAspect, a tracecut specifies a program property as a regular expression. At runtime, there is a tracecut monitor, which is modeled as a finite-state machine, that detects whenever the tracecut regular expression matches some sequence of program events.
It is possible to independently monitor the same property on multiple program objects by parameterizing the tracecut. The tracecut engine spawns a new monitor instance for each distinct set of parameters. For example, you could design a tracecut that creates a monitor instance for each FILE object, as is done in the fclose_tracecut sample tracecut plug-in.
The easiest way to define a tracecut is by first defining all the parameters, and then adding call symbols with tc_declare_call_symbol(). Each symbol represents a particular program event (a call to a specified function) that you can reference in a regular expression rule.
When you compile a target program with a tracecut plug-in, you must also link the target program with the tracecut monitoring engine library, -ltracecut
.
When a tracecut rule matches, the monitoring engine calls the tracecut advice function, tc_report_match(), which prints a message to stderr
by default. The tc_report_match() function is defined as a weak symbol, so you can override it by defining your own version.
Tracecuts are available starting with InterAspect version 1.1.
Function Documentation
enum tc_error tc_add_call_symbol | ( | struct tc_tracecut * | tc, | |
const char * | name, | |||
const char * | func_name, | |||
enum aop_insert_location | location | |||
) |
Add a new tracecut symbol representing a function call event. The event bound to this new symbol is triggered whenever the specified function is called.
All symbols must have unique name: attempting to create a duplicate symbol will fail. It is possible to create multiple symbols for the same function.
(Since version 1.1)
- Parameters:
-
tc The tracecut to add a symbol to. name The name used to reference this symbol. func_name The name of the function that should trigger the new symbol's event. location Specifies whether the the symbol event should trigger before or after the specified function is called.
- Returns:
- TC_SUCCESS or
- TC_BAD_CONTEXT, when called after compilation has already started;
- TC_DUPLICATE, if there already exists a symbol with the specified name;
- TC_BAD_ID if name is not an acceptable identifier. (Identifiers must only contain letters, numbers and the underscore character, with no spaces. Identifiers are case sensitive and should be no longer than TC_MAX_ID_SIZE.)
- TC_NOMEM, if memory runs out.
enum tc_error tc_add_param | ( | struct tc_tracecut * | tc, | |
const char * | name, | |||
const struct aop_type * | type | |||
) |
Add a new tracecut parameter. Each tracecut tracks several objects, so a tracecut event must be parameterized to specify which runtime object it applies to. Use of of tracecut's bind functions to bind a tracecut param to an actual runtime value captured by InterAspect.
All params must have an aop_type that matches pointer types only (i.e., aop_is_pointer_type() returns true).
(Since version 1.1)
- Parameters:
-
tc The tracecut to add a param to. name The name used to reference this param. type They type of this param.
- Returns:
- TC_SUCCESS or
- TC_BAD_CONTEXT, when called after compilation has already started;
- TC_DUPLICATE, if there already exists a param with the specified name;
- TC_BAD_ID if name is not an acceptable identifier. (Identifiers must only contain letters, numbers and the underscore character, with no spaces. Identifiers are case sensitive and should be no longer than TC_MAX_ID_SIZE.)
- TC_INVAL, if aop_is_pointer_type() is false for the specified type;
- TC_NOMEM, if memory runs out.
- Examples:
- fclose_tracecut.c.
enum tc_error tc_add_rule | ( | struct tc_tracecut * | tc, | |
const char * | specification | |||
) |
Add a rule to a tracecut, specified as a regular expression. A tracecut regular expression can include any of the tracecut's symbols (expressed with symbol_name
strings) combined with the standard |
, *
, +
, and ?
operators and parenthesis for grouping.
(Since version 1.1)
- Parameters:
-
tc The tracecut to add the rule to. specification A regular expression over the alphabet of the tracecut's symbols.
- Returns:
- TC_SUCCESS or
- TC_BAD_CONTEXT, when called after compilation has already started;
- TC_NOMEM, if memory runs out.
- Examples:
- fclose_tracecut.c.
enum tc_error tc_bind_to_call_param | ( | struct tc_tracecut * | tc, | |
const char * | param_name, | |||
const char * | symbol_name, | |||
int | call_param_index | |||
) |
Bind a tracecut param to a call symbol's call_param
. Binding to a call_param
will capture that parameter at runtime.
(Since version 1.1)
- Parameters:
-
tc The tracecut to add a binding to. param_name Name of the tracecut param to bind. symbol_name Name of the call symbol to bind to. call_param_index Index of the call_param
to bind to (starting from 0).
- Returns:
- TC_SUCCESS or
- TC_BAD_CONTEXT, when called after compilation has already started;
- TC_INVAL, if call_param_index is negative;
- TC_NOENT, if either the symbol or the binding does not exist;
- TC_NOMEM, if memory runs out.
enum tc_error tc_bind_to_return_value | ( | struct tc_tracecut * | tc, | |
const char * | param_name, | |||
const char * | symbol_name | |||
) |
Bind a tracecut param to a call symbol's return value. Binding to return value will capture that return value at runtime.
(Since version 1.1)
- Parameters:
-
tc The tracecut to add a binding to. param_name Name of the tracecut param to bind. symbol_name Name of the call symbol to bind to.
- Returns:
- TC_SUCCESS or
- TC_BAD_CONTEXT, when called after compilation has already started;
- TC_BAD_RETURN_BINDING, if the specified symbol has a location of AOP_INSERT_BEFORE;
- TC_NOENT, if either the symbol or the binding does not exist;
- TC_NOMEM, if memory runs out.
struct tc_tracecut* tc_create_tracecut | ( | void | ) | [read] |
Create an empty tc_tracecut object. The caller is responsible for freeing the object using tc_free_tracecut(). (Do not use the standard free function.)
(Since version 1.1)
- Returns:
- A new tc_tracecut that must be freed with tc_free_tracecut(). The return value will be NULL if allocation fails or if compilation has already started..
- Examples:
- fclose_tracecut.c.
enum tc_error tc_declare_call_symbol | ( | struct tc_tracecut * | tc, | |
const char * | name, | |||
const char * | declaration, | |||
enum aop_insert_location | location | |||
) |
Create a function call symbol along with its bindings in one statement. The declaration is a string containing the function name and all the bindings in a special format. To bind the first and second call parameters, use the format:
"func_name(param1, param2)"
Use ?
to skip a call parameter. To bind only the second call parameter, for example:
"func_name(?, param)"
To bind the return value:
"(param)func_name()"
(Since version 1.1)
- Parameters:
-
tc The tracecut to add the symbol to. name The name used to reference this symbol. declaration A string declaring the name of the function that should trigger this symbol's event, as well as which param bindings to add. location Specifies whether the the symbol event should trigger before or after the specified function is called.
- Returns:
- TC_SUCCESS or
- TC_BAD_CONTEXT, when called after compilation has already started;
- TC_INVAL, when the declaration is invalid because of syntax errors;
- TC_DUPLICATE, if there already exists a symbol with the specified name;
- TC_BAD_ID if name is not an acceptable identifier. (Identifiers must only contain letters, numbers and the underscore character, with no spaces. Identifiers are case sensitive and should be no longer than TC_MAX_ID_SIZE.)
- TC_BAD_RETURN_BINDING, if the the declaration binds the return value but the location is AOP_INSERT_BEFORE;
- TC_NOENT, if one of the parameters in the declaration does not exist;
- TC_NOMEM, if memory runs out.
- Examples:
- fclose_tracecut.c.
enum tc_error tc_error_code | ( | struct tc_tracecut * | tc | ) |
The first operation on a tracecut that fails stores its error code in the tracecut. This function returns that error code, or TC_SUCCESS if all operations succeeded. Checking error codes this ways makes it possible to call several tracecut functions in sequence without individually checking their error codes.
Use tc_reset_error() to set a tracecut's error code back to TC_SUCCESS.
(Since version 1.1)
- Parameters:
-
tc The tracecut to check.
- Returns:
- The error code of the first failed operation on this tracecut, or TC_SUCCESS if no operations failed.
- Examples:
- fclose_tracecut.c.
void tc_free_tracecut | ( | struct tc_tracecut * | tc | ) |
Free all the memory used by a tc_tracecut object. Every call to tc_create_tracecut() should have a matching call to tc_free_tracecut().
(Since version 1.1)
- Parameters:
-
tc The tracecut object to free.
- Examples:
- fclose_tracecut.c.
void tc_insert_tracecut_init_advice | ( | struct aop_joinpoint * | jp | ) |
Adds advice calls that initialize the tracecut runtime. You will always almost want to use tc_set_main_function(), which automatically adds the tracecut initialization advice, instead of this function.
If you do want to manually add tracecut initialization, you must create your own InterAspect pass that finds a join point where initialization should go. This join point should execute only once, right when you want tracecut monitoring to start.
(Since version 1.1)
- Parameters:
-
jp The join point to insert initialization at.
void tc_register_tracecut_pass | ( | void | ) |
Call this from your aop_main() function to register a pass that will add tracecut instrumentation. A tracecut plug-in will not do anything without this call!
(Since version 1.1)
- Examples:
- fclose_tracecut.c.
void tc_reset_error | ( | struct tc_tracecut * | tc | ) |
Clear the history of any failed operations on this tracecut. After calling this, tc_error_code() will return TC_SUCCESS until some later tracecut operation fails.
(Since version 1.1)
- Parameters:
-
tc The tracecut to reset.
void tc_set_main_function | ( | const char * | func_name | ) |
Specifies the name of the target program's main function. The main function should be a function that executes exactly once at the beginning of program execution. Usually, main
is the obvious main function.
The tracecut plug-in will insert tracecut runtime initialization code at the entry to the main function. For tracecut monitoring to work, you must either set a main function or manually add this initiliazation code using tc_insert_tracecut_init_advice().
(Since version 1.1)
- Parameters:
-
func_name The name of the main function.
- Examples:
- fclose_tracecut.c.
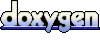