InterAspect Types
Function Documentation
void aop_cast_to_all_fp | ( | struct aop_dynval * | dv | ) |
Modify an aop_dynval object so that it will be passed to advice as if it were matched and captured using the aop_t_all_fp() type.
Only use this function on dynvals whose type passes aop_is_all_fp_subtype(). Casting a non-float type with this function will raise a fatal compiler error.
(Since version 1.1)
- Parameters:
-
dv The aop_dynval to cast.
void aop_cast_to_all_pointer | ( | struct aop_dynval * | dv | ) |
Modify an aop_dynval object so that it will be passed to advice as if it were matched and captured using the aop_t_all_pointer() type. Casting is useful if you wish to capture values from pointcuts matched with different aop_type objects but pass those values to the same advice function.
Only use this function on dynvals whose type passes aop_is_pointer_type(). Casting a non-pointer type with this function will raise a fatal compiler error.
(Since version 1.1)
- Parameters:
-
dv The aop_dynval to cast.
void aop_cast_to_all_signed | ( | struct aop_dynval * | dv | ) |
Modify an aop_dynval object so that it will be passed to advice as if it were matched and captured using the aop_t_all_signed() type.
Only use this function on dynvals whose type passes aop_is_all_signed_subtype(). Casting any other type with this function will raise a fatal compiler error.
(Since version 1.1)
- Parameters:
-
dv The aop_dynval to cast.
void aop_cast_to_all_unsigned | ( | struct aop_dynval * | dv | ) |
Modify an aop_dynval object so that it will be passed to advice as if it were matched and captured using the aop_t_all_unsigned() type.
Only use this function on dynvals whose type passes aop_is_all_unsigned_subtype(). Casting any other type with this function will raise a fatal compiler error.
(Since version 1.1)
- Parameters:
-
dv The aop_dynval to cast.
struct aop_type* aop_get_dynval_type | ( | struct aop_dynval * | dv | ) | [read] |
Get the type for an aop_dynval object. Every dynval has an associated type that is determined by how it was matched and captured.
(Since version 1.1)
- Returns:
- The type of the specified dynval.
int aop_is_all_fp_subtype | ( | const struct aop_type * | type | ) |
Check if an aop_type object matches one of the types that aop_t_all_fp() matches.
(Since version 1.1)
- Parameters:
-
type The type to check.
- Returns:
- Non-zero if the aop_type object is standard-sized signed floating point type.
int aop_is_all_signed_subtype | ( | const struct aop_type * | type | ) |
Check if an aop_type object matches one of the types that aop_t_all_signed() matches.
(Since version 1.1)
- Parameters:
-
type The type to check.
- Returns:
- Non-zero if the aop_type object is standard-sized signed integer type.
int aop_is_all_unsigned_subtype | ( | const struct aop_type * | type | ) |
Check if an aop_type object matches one of the types that aop_t_all_unsigned() matches.
(Since version 1.1)
- Parameters:
-
type The type to check.
- Returns:
- Non-zero if the aop_type object is standard-sized unsigned integer type.
int aop_is_pointer_type | ( | const struct aop_type * | type | ) |
Check if an aop_type object matches a pointer type. This check is true if and only if the specified type matches a subset of types that aop_t_all_pointer() matches.
(Since version 1.1)
- Parameters:
-
type The type to check.
- Returns:
- Non-zero if the aop_type object matches a pointer type, zero otherwise.
struct aop_type* aop_t_all_fp | ( | ) | [read] |
Return a type that will match any standard-sized floating point type.
Standard floating point sizes are single-precision (float) and double-precision (double). This type will not match higher-precision floating point types (you must use aop_t_long_double() to match GCC's long double).
- Returns:
- A type that will match any standard-sized floating point type.
struct aop_type* aop_t_all_pointer | ( | ) | [read] |
Return a type that will match any pointer.
- Returns:
- A type that will match any pointer.
- Examples:
- fclose_tracecut.c.
struct aop_type* aop_t_all_signed | ( | ) | [read] |
Return a type that will match any standard-sized signed integer type.
Standard integer sizes are 1, 2, 4, and 8 bytes. This type will not match 16-byte or larger integer types (you must use aop_t_signed128() for 16-byte signed integers).
When passing an aop_t_all_signed() value to an advice function (via aop_insert_advice()) the value is automatically cast to the (long long int) type, which is guaranteed by the C standard to be large enough to hold any standard-sized integer.
Be careful: (long long int) is larger than (int) on most platforms. We recommend defining advice functions using the ALL_SIGNED_T macro to avoid confusion.
- Returns:
- A type that will match any standard-sized signed integer type.
- Examples:
- advice_header.c, and duplicate.c.
struct aop_type* aop_t_all_unsigned | ( | ) | [read] |
Return a type that will match any standard-sized unsigned integer type.
Standard integer sizes are 1, 2, 4, and 8 bytes. This type will not match 16-byte or larger integer types (you must use aop_t_unsigned128() for 16-byte unsigned integers).
When passing an aop_t_all_unsigned() value to an advice function (via aop_insert_advice()) the value is automatically cast to the (long long unsigned) type, which is guaranteed by the C standard to be large enough to hold any standard-sized integer.
Be careful: (long long unsigned) is larger than unsigned (int) on most platforms. We recommend defining advice functions using the ALL_UNSIGNED_T macro to avoid confusion.
- Returns:
- A type that will match any standard-sized unsigned integer type.
struct aop_type* aop_t_cstring | ( | ) | [read] |
A convenience function for getting a (char *)
type. This is the same as passing aop_t_signed8() to aop_t_pointer_to().
- Returns:
- A type that will match the type
(char *)
.
struct aop_type* aop_t_enum | ( | const char * | tag | ) | [read] |
Return a type that will match an enum type. Note that this will not match pointers to the specified enum type.
- Parameters:
-
tag The name of the enum. To match enum foo
, give justenum
as the tag.
- Returns:
- A type that will match a specified union.
struct aop_type* aop_t_float32 | ( | ) | [read] |
Return a type that will match single-precision floating point types (float in C).
- Returns:
- A type that will match single-precision floating point types.
struct aop_type* aop_t_float64 | ( | ) | [read] |
Return a type that will match double-precision floating point types (double in C).
- Returns:
- A type that will match double-precision floating point types.
struct aop_type* aop_t_long_double | ( | ) | [read] |
Return a type that will match long double
. Where supported, the long double
type is actually 80-bits, though it is padded to 96 or 128 bits on different systems.
- Returns:
- A type that will match GCC's
long double
floating point type.
Return a type that will match pointers to the specified type.
NB: It is possible to match pointers to aop_t_all_signed(), aop_t_all_unsigned(), or aop_t_all_fp(), but it is dangerous to then capture the matched value and pass it to an advice function. The advice function will have no way of knowing which type was matched. For example, if an advice function gets a pointer to an "all fp" value, should it dereference it as a double or a float?
- Returns:
- A type that will match pointers to the specified type.
- Examples:
- advice_header.c.
struct aop_type* aop_t_signed128 | ( | ) | [read] |
Return a type that will match any 128-bit signed integer (__int128_t on platforms that support 128-bit integers).
- Returns:
- A type that will match 128-bit signed integers.
struct aop_type* aop_t_signed16 | ( | ) | [read] |
Return a type that will match any 16-bit signed integer (int16_t or, on most platforms, short).
- Returns:
- A type that will match 16-bit signed integers.
struct aop_type* aop_t_signed32 | ( | ) | [read] |
Return a type that will match any 32-bit signed integer (int32_t or, on most platforms, int).
- Returns:
- A type that will match 32-bit signed integers.
struct aop_type* aop_t_signed64 | ( | ) | [read] |
Return a type that will match any 64-bit signed integer (int64_t or, on most platforms, long long int).
- Returns:
- A type that will match 64-bit signed integers.
struct aop_type* aop_t_signed8 | ( | ) | [read] |
Return a type that will match any 8-bit signed integer (such as char or int8_t).
- Returns:
- A type that will match 8-bit signed integers.
- Examples:
- advice_header.c.
struct aop_type* aop_t_struct | ( | const char * | tag | ) | [read] |
Return a type that will match a struct type. Note that this will not match pointers to the specified struct type.
- Parameters:
-
tag The name of the struct. To match struct foo
, give justfoo
as the tag.
- Returns:
- A type that will match a specified struct.
struct aop_type* aop_t_struct_ptr | ( | const char * | tag | ) | [read] |
A convenience function for obtaining a type that will match a pointer to a specified struct. The result is the same as obtaining a struct type with aop_t_struct() and passing it to aop_t_pointer_to().
- Parameters:
-
tag The name of the struct. To match struct foo *
, give justfoo
as the tag.
- Returns:
- A type that will match pointers to a specified struct.
struct aop_type* aop_t_union | ( | const char * | tag | ) | [read] |
Return a type that will match a union type. Note that this will not match pointers to the specified union type.
- Parameters:
-
tag The name of the union. To match union foo
, give justfoo
as the tag.
- Returns:
- A type that will match a specified union.
struct aop_type* aop_t_union_ptr | ( | const char * | tag | ) | [read] |
A convenience function for obtaining a type that will match a pointer to a specified union. The result is the same as obtaining a union type with aop_t_union() and passing it to aop_t_pointer_to().
- Parameters:
-
tag The name of the union. To match union foo *
, give justfoo
as the tag.
- Returns:
- A type that will match pointers to a specified union.
struct aop_type* aop_t_unsigned128 | ( | ) | [read] |
Return a type that will match any 128-bit unsigned integer (__uint128_t on platforms that support 128-bit integers).
- Returns:
- A type that will match 128-bit unsigned integers.
struct aop_type* aop_t_unsigned16 | ( | ) | [read] |
Return a type that will match any 16-bit unsigned integer (uint16_t or, on most platforms, unsigned short).
- Returns:
- A type that will match 16-bit unsigned integers.
struct aop_type* aop_t_unsigned32 | ( | ) | [read] |
Return a type that will match any 32-bit unsigned integer (uint32_t or, on most platforms, unsigned int).
- Returns:
- A type that will match 32-bit unsigned integers.
struct aop_type* aop_t_unsigned64 | ( | ) | [read] |
Return a type that will match any 64-bit unsigned integer (uint64_t or, on most platforms, long long unsigned).
- Returns:
- A type that will match 64-bit unsigned integers.
struct aop_type* aop_t_unsigned8 | ( | ) | [read] |
Return a type that will match any 8-bit unsigned integer (such as unsigned char or uint8_t).
- Returns:
- A type that will match 8-bit unsigned integers.
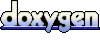