Advice Insertion Functions
Defines | |
#define | AOP_STR_CST(VAL) ATA_STR_CST, VAL |
Mark an advice argument as a string constant (const char *). | |
#define | AOP_INT_CST(VAL) ATA_INT_CST, VAL |
Mark an advice argument as an integer constant (int). | |
#define | AOP_DOUBLE_CST(VAL) ATA_DOUBLE_CST, VAL |
Mark an advice argument as a floating-point constant (double). | |
#define | AOP_VOIDP_CST(VAL) ATA_VOIDP_CST, VAL |
Mark an advice argument as an void pointer constant (void *). | |
#define | AOP_DYNVAL(VAL) ATA_DYNVAL, VAL |
Mark an advice argument as an runtime value (struct aop_dynval *). | |
#define | AOP_TERM_ARG |
End a list of advice arguments. | |
Enumerations | |
enum | aop_insert_location { AOP_INSERT_BEFORE, AOP_INSERT_AFTER } |
Advice locations for aop_insert_advice(). More... | |
Functions | |
void | aop_insert_advice (struct aop_joinpoint *jp, const char *func_name, enum aop_insert_location location,...) |
void | aop_duplicate (struct aop_joinpoint *jp, const char *func_name,...) |
Define Documentation
#define AOP_DOUBLE_CST | ( | VAL | ) | ATA_DOUBLE_CST, VAL |
Mark an advice argument as a floating-point constant (double).
Use this macro when passing a floating-point constant (double) argument to aop_insert_advice(). Make sure the argument actually has type double. The preprocessor cannot type check this input, and an input with the wrong type (such as float) can cause a memory error.
#define AOP_DYNVAL | ( | VAL | ) | ATA_DYNVAL, VAL |
Mark an advice argument as an runtime value (struct aop_dynval *).
Use this macro when passing a runtime value (struct aop_dynval *) argument to aop_insert_advice().
- Examples:
- advice_header.c, and duplicate.c.
#define AOP_INT_CST | ( | VAL | ) | ATA_INT_CST, VAL |
Mark an advice argument as an integer constant (int).
Use this macro when passing an integer constant (int) argument to aop_insert_advice(). Make sure the argument actually has type int, and not a different-sized type (like long on a 64-bit system). The preprocessor cannot type check this input, and an input with the wrong type can cause a memory error.
#define AOP_STR_CST | ( | VAL | ) | ATA_STR_CST, VAL |
Mark an advice argument as a string constant (const char *).
Use this macro when passing a string constant (const char *) argument to aop_insert_advice().
- Examples:
- duplicate.c, and hello.c.
#define AOP_TERM_ARG |
End a list of advice arguments.
This value must always be the last thing passed to aop_insert_advice() and aop_duplicate(), even if you are not passing any advice arguments.
- Examples:
- advice_header.c, duplicate.c, and hello.c.
#define AOP_VOIDP_CST | ( | VAL | ) | ATA_VOIDP_CST, VAL |
Mark an advice argument as an void pointer constant (void *).
Use this macro when passing an void pointer constant (void *) argument to aop_insert_advice().
Enumeration Type Documentation
enum aop_insert_location |
Advice locations for aop_insert_advice().
Specifying AOP_INSERT_BEFORE or AOP_INSERT_AFTER will place the call before or after the join point (when possible) and will guarantee certain properties about ordering of advice calls. Using AOP_INSERT_BEFORE to insert multiple advice calls at the same join point will place advice calls in the order they are inserted. With AOP_INSERT_AFTER, however, advice calls will be placed in _reverse_ order. No matter what order calls are inserted in, all AOP_INSERT_BEFORE calls will be placed before all AOP_INSERT_AFTER calls.
It is legal to use AOP_INSERT_BEFORE and AOP_INSERT_AFTER to enforce ordering among advice calls even when inserting before or after a join point does not make sense. For example, it does not make sense to insert an advice call before a function entry join point. Using AOP_INSERT_BEFORE will still insert the advice after function entry, but it will place the advice before any AOP_INSERT_AFTER advice.
- Enumerator:
AOP_INSERT_BEFORE Insert advice before a join point when possible.
Even when it does not make sense to insert advice before a join point, all AOP_INSERT_BEFORE advice will be ordered before all AOP_INSERT_AFTER advice.
If multiple advice calls are inserted with AOP_INSERT_BEFORE, they will be placed in the order they were inserted.
AOP_INSERT_AFTER Insert advice after a join point when possible.
Even when it does not make sense to insert advice before a join point, all AOP_INSERT_BEFORE advice will be ordered before all AOP_INSERT_AFTER advice.
If multiple advice calls are inserted with AOP_INSERT_AFTER, they will be placed in the reverse of the order they were inserted in.
Function Documentation
void aop_duplicate | ( | struct aop_joinpoint * | jp, | |
const char * | func_name, | |||
... | ||||
) |
Duplicate the current function's body so that there are two copies available for instrumentation. A function entry join point is required because the duplication process inserts an advice call at function entry which serves as a distributor.
The distributor advice decides which copy to execute. A distributor advice function returns an int (unlike regular advice, which has void return type): a zero value will execute the original copy and a non-zero value will execute the new copy.
Any arguments following func_name are arguments to be passed to the distributor advice function. (See the documentation for aop_insert_advice() for an explanation of passing arguments to advice.) AOP_TERM_ARG must be the last argument to aop_duplicate(), even when not specifying any arguments for the advice function.
See the duplicate.c sample plug-in for a simple example of function duplication.
- Parameters:
-
jp The function entry join point for the current join point. The distributor advice will be inserted here. Function entry join points are obtained by joining on an aop_match_function_entry() pointcut. func_name The name of the distributor advice function. ... A list of arguments to pass to the advice function, terminated by AOP_TERM_ARG.
- Examples:
- duplicate.c.
void aop_insert_advice | ( | struct aop_joinpoint * | jp, | |
const char * | func_name, | |||
enum aop_insert_location | location, | |||
... | ||||
) |
Insert a call to an advice function at a join point with any number of arguments. Any arguments following location are arguments to be passed to the advice function. Because C does not know the types of vararg arguments, a type macro (such as AOP_STR_CST or AOP_DYNVAL) is necessary for each advice argument. AOP_TERM_ARG must be the last argument to aop_insert_advice(), even when not specifying any arguments for the advice function.
- Parameters:
-
jp The join point to instrument with advice. func_name The name of the advice function. location Where to insert advice with respect to the join point. See the documenation for enum aop_insert_location for more detail. ... A list of arguments to pass to the advice function, terminated by AOP_TERM_ARG.
- Examples:
- advice_header.c, duplicate.c, and hello.c.
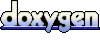